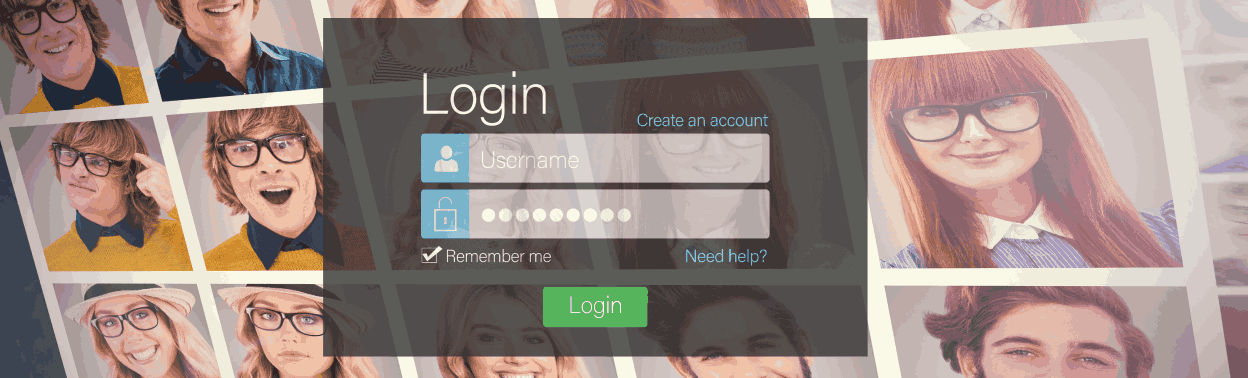
Why add social login to your app?
There are several benefits of adding social login to your mobile app:
- Improved user experience: Users no longer have to remember any passwords and type long login-Id’s or emails. Therefore helping them save time and effort logging into your application, Simplifying the sign-up and login process for better user acquisition.
- Access to user data: When a user signups using social media, we can request and access certain data from their social media profiles.This data can help us better understand your users.
- Trust and security: Adding social login to your application provides an added layer of security for user authentication. This can help prevent fraudulent sign-ups and increase the safety of user accounts.
- Saved development time: Implementing social login in your app saves you from building a custom authentication system, Which can also help in a lot of resources saved.
Now that you know some of the benefits of using social login in your applications, let’s start!
- How to add google and facebook login to your flutter application and use it to save and create a user.
- Adding Social media auth as firebase function.
- By the end of this tutorial, readers will have gained the knowledge and practical experience needed to integrate social login into their Flutter applications, enhancing user authentication and overall app functionality.
Things You Need
- Google and Facebook Accounts
- Basic Understanding of dart language and Flutter framework
- An IDE like VSCODE or Android Studio
Google Login
Set up your Flutter project:
First, make sure you have Flutter SDK installed and configured on your machine. If not, you can follow the official Flutter installation guide here: https://docs.flutter.dev/get-started/install
To set up a new Flutter project, run the following command in your terminal:
Step 1:
flutter create firebase_google_login
Now open the project in VS Code or Android Studio.
Step 2:
Add the required dependencies in your project
Open the pubspec.yaml file in your project and add the following dependencies:
dependencies:
flutter:
sdk: flutter
firebase_core: ^2.23.0
firebase_auth:^4.14.1
google_sign_in: ^6.1.6
Make sure you change the package version to the latest by going to pub.dev and looking for possible version updates.
Now, save the pubsepc.yaml file and run “flutter pub get” in the terminal to fetch the required packages.
Step 3:
Set up Firebase project
Goto to your browser and open Firebase Console (console.firebase.google.com) and create a new project. Follow the instructions to set up your project, including enabling required authentication providers such as Google and Facebook.
You just need to goto authentication tab and enable the required google login.
But to be able to use google authentication, you need a SHA key for your project, you can follow the instructions given on the firebase console.
For Android, you need an SHA key, which you can easily generate in your IDE, by typing the following command in terminal:
keytool -list -v \ -alias androiddebugkey -keystore ~/.android/debug.keystore
Hit Enter after typing and type the default password : “android” and you will get a generated SHA key.
Now goto your project settings, and paste the SHA key by selecting your android project, You can add both keys SHA-1 and SHA-256.
All the necessary instructions will be provided on screen which you can easily follow and set up.
Now let’s move to our project.
Enable google from this screen
Step 4:
Initialize Firebase in your Flutter app
Now, goto your main.dart file in the project, import the necessary Firebase and authentication packages:
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_auth/firebase_auth.dart';
import 'package:google_sign_in/google_sign_in.dart';
Then, initialize Firebase in the main method before calling runApp():
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
Step 5:
Implementing the google login functionality and setting gradle.properties file
Add following lines to your “gradle.properties” file
android.useAndroidX=true
android.enableJetifier=true
Finally make a new file named database_methods.dart. And write a function “addUserDetails()” which will take the userdetails and map them to the firebase cloud Firestore database.
defaultConfig {
multiDexEnabled true}
Create a new Dart file called auth.dart for handling auth logic.
Make sure to Import FirebaseAuth and GoogleSignIn:
import 'package:firebase_auth/firebase_auth.dart';
import 'package:google_sign_in/google_sign_in.dart';
Create a FirebaseAuth instance:
final FirebaseAuth auth = FirebaseAuth.instance;
Declare a variable to hold the current user:
User? user;
Now let’s Define a method to sign in with Google:
Future signInWithGoogle() async {
final GoogleSignInAccount? googleUser = await GoogleSignIn().signIn();
final GoogleSignInAuthentication googleAuth =
await googleUser!.authentication;
final OAuthCredential credential = GoogleAuthProvider.credential(
accessToken: googleAuth.accessToken,
idToken: googleAuth.idToken,
);
return await auth.signInWithCredential(credential);
}
To understand let’s break down this function:
This line starts the flow for the Sign-In instance
final GoogleSignInAccount? googleUser = await GoogleSignIn().signIn();
This line gets the user’s authentication details and save to googleAuth instance
final GoogleSignInAuthentication googleAuth =
await googleUser!.authentication;
This line creates a credential from the Google Sign-In authentication
final OAuthCredential credential = GoogleAuthProvider.credential(
accessToken: googleAuth.accessToken,
idToken: googleAuth.idToken,
);
This line signs the user in to Firebase using the Google OAuthCredential
return await auth.signInWithCredential(credential);
Finally, define a method to sign out from google.
Future signOut() async {
await GoogleSignIn().signOut();
await auth.signOut();
}
Step 6:
Now let’s make a user interface for the project.
Login_screen.dart
class LoginScreen extends StatelessWidget {
const LoginScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Login Screen'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
Auth().signInWithGoogle().then((userCredential) {
//take user to home screenNavigator.of(context).pushReplacement(
Navigator.of(context).pushReplacement(
MaterialPageRoute(
builder: (context) => const HomePage(),
),
);
// Handle successful sign-in
}).catchError((error) {
// Handle sign-in error
});
},
child: const Text('Sign in with Google'),
),
),
);
}
}
Homepage.dart
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Home Page'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text(
'Welcome!',
style: TextStyle(
fontSize: 24,
fontWeight: FontWeight.bold,
),
),
const SizedBox(height: 16),
const Text(
'You are logged in with Google.',
style: TextStyle(
fontSize: 16,
),
),
const SizedBox(height: 16),
ElevatedButton(
onPressed: () async {
await GoogleSignIn().disconnect();
FirebaseAuth.instance.signOut();
Navigator.of(context).pushReplacement(
MaterialPageRoute(
builder: (context) => const LoginScreen(),
),
);
},
child: const Text('Logout'),
),
],),
),);
}}
Here we are simply showing a simple button on the LoginScreen, which calls “signInWithGoogle” method and the user is shown a modal on screen, where the user has to select the desired account and upon selection.
A new user is created (which you can see from the firebase console) and then the user is taken to the next screen that shows the user is successfully created and if you take a look at the code we are calling the logout method, which log-outs the current signed in user.
Screenshots:
This simple screen is the entry point, where users can login using their google account, by tapping the “Sign In with Google” button.
From this screen you can select the desired account and tap on it.
If the user is successfully created, The screen navigates to this “HomeScreen” where you can perform any actions you want. Currently we are just showing a logout button, which logs-out the current user and takes us to the login page.
Facebook Login
Now that you have learned how to add Google Authentication for Login, let’s move to facebook and configure our project for Facebook Signup-Login.
Step 1:
Update pubspec.yaml file to add following package:
Flutter_facebook_auth
dependencies:
flutter:
sdk: flutter
firebase_core: ^2.23.0
flutter_facebook_auth: ^6.0.3 //ADD THIS PACKAGE <===
firebase_auth:^4.14.1
google_sign_in: ^6.1.6
And save your pubspec.yaml file.
Remember, not to run the project just now, First complete all the steps and then go ahead.
Step 2:
Goto your android project:
android/app/build.gradle
Make sure minSdkVersion is set to at least 2
Step 3:
Go to Facebook Login for Android – Quickstart, and follow the instructions after login to your account.
Follow the flow from these screenshots:
Now Tap on the “Create App” button.
Step 4:
Add login with facebook button and update the auth file for facebook login, Update the files by adding a new elevated button with following on-pressed function and a new function for facebook login.
login_screen.dart
ElevatedButton(
onPressed: () {
debugPrint('Sign in with Facebook');
Auth().signInWithFacebook().then((userCredential) {
//take user to home screenNavigator.of(context).pushReplacement(
Navigator.of(context).pushReplacement(
MaterialPageRoute(
builder: (context) => const HomePage(
loginMethod: 'Facebook',
),
),
);
// Handle successful sign-in
}).catchError((error) {
error.toString();
// Handle sign-in error
});
},
child: const Text('Sign in with Facebook'),
),
Auth.dart
//write function for facebook login
Future signInWithFacebook() async {
// Trigger the sign-in flow
debugPrint('Sign in with Facebook here im');
var result = await FacebookAuth.instance.login();
debugPrint('Sign in with Facebook here im 2 = $result');
if (result.status == LoginStatus.failed) {
print(result.status);
print(result.message);
}
// Create a credential from the access token
final OAuthCredential facebookAuthCredential =
FacebookAuthProvider.credential(result.accessToken!.token);
// Once signed in, return the UserCredential
return await FirebaseAuth.instance
.signInWithCredential(facebookAuthCredential);
}
Now It’s time to Edit Your Resources and Manifest add this config in your android project.
/android/app/src/main/res/values/strings.xml
Got to above location in you project, If strings.xml file does not exist,
Make sure you make one.
Now, add the following to the file,Add your own app id here.
DDE(TH$LW
YOUR_CLIENT_TOKEN
You can find the AppId and client token here:
Step 5:
Update AndroidManifest.xml
Add Internet permission by adding the following, before the application element:
Now, in the the same file, inside application element add following:
These 2 lines of code, add an activity for Facebook and an activity and intent filter for Chrome Custom Tabs.
Now, we will associate the “Package Name” and “Default Class” with our Application project.
Both of which you can find in the same file AndroidManifest.xml.
Now add this line under the <manifest> tag, this will use the facebook app if it’s available and installed on your phone.
Step 6:
Associating Package Name and Default Class with the App
goto: Android – Facebook Login – Documentation – Meta for Developers
Enter the package name and default activity class name from the “AndroidManifest.xml” file and save.
Now, to provide the key hashes as they are required, use the following commands based on your system:
MacOS:
keytool -exportcert -alias androiddebugkey -keystore ~/.android/debug.keystore | openssl sha1 -binary | openssl base64
Windows:
keytool -exportcert -alias androiddebugkey -keystore "C:\Users\USERNAME\.android\debug.keystore" | "PATH_TO_OPENSSL_LIBRARY\bin\openssl" sha1 -binary | "PATH_TO_OPENSSL_LIBRARY\bin\openssl" base64
Enable the following option, If you would like your Android Notifications to have the ability to launch your app, enable single sign on.
One thing to keep in mind is that you keep the intent outside the <activity> tag in the “AndroidManifest.xml” file, otherwise you may run into issues.
Great! You have completed all things necessary for using Facebook login as a method. Let’s run the application now.
We have successfully created our facebook Auth user inside firebase.
As you can see our user is generated and on successful login, the user is taken to the homepage.
Conclusion
Today you learned how easy it is to add social login to your flutter application and we learned how to configure our project to support both google and facebook login.
You first started out with a default flutter project, then you learned how to build a User Interface that is simple and clear, and then you wrote the functions for google and facebook auth and finally how to logout.
If this article has helped in any way and you have learned something new today, Kindly consider sharing the article so others can also benefit and learn how to build simple AI applications.
Subscribe to our website for more interesting articles on AI and other interesting fields of IT.
You can find the code for this here:
Repository Link:
- Repo link: github