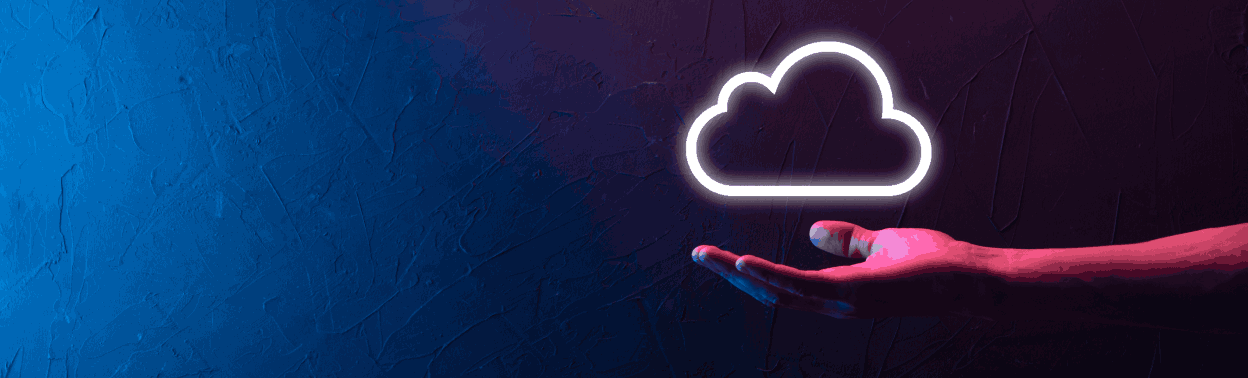
Introduction
Firebase Cloud Messaging (FCM) is a service provided by firebase that allows us to send notifications and messages to multiple platforms, without any cost.
This service is multiplatform, meaning that it not only supports Android or IOS but now you can use this service to send notifications to all Web as well.
FCM allows us to send notifications, data messages, or a combination of both.
Firebase Cloud Messaging allows us to receive notifications while the app is in the foreground, in the background, and even if the app is killed.
Benefits of using Firebase FCM
Your app can benefit from using FCM:
- User Engagement: You can send notifications about new features, content, or promotions to engage your users and bring them back to your app.
- Real-time Updates: If your app involves real-time data (like a chat app or a sports score app), you can use FCM to notify users of updates.
- Reliability: FCM is reliable and optimized for performance. It handles message routing and delivery to targeted devices.
- Versatility: You can send messages to single devices, to groups of devices, or to devices subscribed to topics.
- Payload: You can send up to 4KB of payload with your messages, which can include images, sounds, badges, and other options.
Here’s a simple example of how to send a notification message using FCM:
Let’s understand with a example
We will create a simple application in Flutter which will show notifications using the firebase console notification composer.
Now that you know what we are going to build, I want you to focus and understand each step as this will help you understand how state and cubit work together to update the UI.
Things You Need
- Basic Understanding of Flutter Framework
- IDE like VSCODE or Android Studio
- Mobile device for testing
Getting Started
Set up your Flutter project:
First, make sure you have Flutter and Dart installed on your machine. If not, you can follow the official Flutter installation guide here: https://docs.flutter.dev/get-started/install
Create a new Flutter project using the following command in your VS code Terminal:
flutter create firebase_notifications_using_fcm , This will create a project for you, Now follow the rest of the instructions.
Define the structure of your project
Setup your firebase console project
Goto https://console.firebase.google.com/ and create a new project.
Write your project name and press continue on next screen as well:
Now it’s time to add firebase to your local project, using firebase CLI
https://console.firebase.google.com/u/
0/project/fir-fcm-3d9fe/messaging/onboarding//
Goto: Project Settings and add you flutter app:
From there follow a simple step by step process to use Firebase CLI and register your project.
This will add necessary configurations for your project.
Add required package
Let’s first add some required packages to our project:
In your terminal run this command ‘flutter pub add firebase_messaging’
and ‘flutter pub add firebase_core’
This will add firebase_messaging and firebase_core packages to your ’pubspec.yaml’ file
You may need to update these packages to the latest versions, as these packages are regularly updated. Find these packages on pub.dev
Configuring Project
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_push_notifications_using_fcm/firebase_options.dart';
import 'package:flutter/material.dart';
Future main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
runApp(const MainApp());
}
class MainApp extends StatelessWidget {
const MainApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: Scaffold(
body: Center(
child: Text('Hello World!'),
),
),
);
}
}
Update your main.dart file as above, Now let’s understand this code:
Here we are importing firebase_core and firebase_options and then on the app run It initializes a new Firebase application instance and DefaultFirebaseOptions.currentPlatform is a set of options that are required for the current platform like Android or IOS.
Request Notification Permissions
Now add this code after Firebase.initializeApp() function ensures the app requests for permissions of notifications on start.
final notificationSettings =
await FirebaseMessaging.instance.requestPermission(provisional: true);
Notifications
In your firebase console open firebase messaging tab and select ‘Create new Campaign’
Now select ‘Firebase Notification Messages’ and select ‘Create’.’
This screen will show up for you to write the notification details.
Enter the title and description and press ‘Next’.’
Select the app you would want to send the notification.
After all the details, press ‘Publish’.’
You have successfully created your first notification for testing:
This is how you will see the notification on your device:
But this notification will only show if your application is in the background. To show a notification to the user whilst the app is in foreground, we have multiple ways. Let’s use a simple toast to display the data of the sent notification using a snackbar.
To display the notification whilst the application is in foreground, we will have to update the code to listen to the notifications and use the data received from notification to build a custom notification using a snackbar to show to the user.
Update the main.dart file as follows:
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_messaging/firebase_messaging.dart';
import 'package:firebase_push_notifications_using_fcm/firebase_options.dart';
import 'package:flutter/material.dart';
final GlobalKey scaffoldMessengerKey =
GlobalKey();
Future main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
final notificationSettings =
await FirebaseMessaging.instance.requestPermission(provisional: true);
String? token = await FirebaseMessaging.instance.getToken();
print('FCM Token: $token');
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
if (message.notification != null) {
final snackBar = SnackBar(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
backgroundColor: Colors.blueGrey[900],
content: ListTile(
leading: const Icon(
Icons.notifications,
color: Colors.white,
),
title: Text(
message.notification!.title!,
style: const TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
subtitle: Text(message.notification!.body!,
style: const TextStyle(color: Colors.white)),
),
duration: const Duration(seconds: 5),
);
scaffoldMessengerKey.currentState?.showSnackBar(snackBar);
}
});
runApp(const MainApp());
}
class MainApp extends StatelessWidget {
const MainApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
scaffoldMessengerKey: scaffoldMessengerKey,
home: const Scaffold(
body: Center(
child: Text('Hello World!'),
),
),
);
}
}
We First added:
final GlobalKey scaffoldMessengerKey =
GlobalKey();
This GlobalKey<ScaffoldMessengerState> allows us to create a global reference to the ScaffoldMessenger state. That gives us access to show SnackBars from anywhere in our code, even outside the widget tree.
scaffoldMessengerKey: scaffoldMessengerKey,
Then add this line under your MaterialApp well. It is used to associate the GlobalKey<ScaffoldMessengerState> with the ScaffoldMessenger of MaterialApp, This is useful in our case, as it will show SnackBar from a Firebase Cloud Messaging callback which doesn’t have access to the widget tree.
String? token = await FirebaseMessaging.instance.getToken();
print('FCM Token: $token');
To get the app token this line retrieves the FCM token for the current app instance. FCM token is a unique identifier that Firebase uses to send messages to this specific app instance.
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
if (message.notification != null) {
final snackBar = SnackBar(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
backgroundColor: Colors.blueGrey[900],
content: ListTile(
leading: const Icon(
Icons.notifications,
color: Colors.white,
),
title: Text(
message.notification!.title!,
style: const TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
subtitle: Text(message.notification!.body!,
style: const TextStyle(color: Colors.white)),
),
duration: const Duration(seconds: 5),
);
scaffoldMessengerKey.currentState?.showSnackBar(snackBar);
}
});
In this code we are listening for Firebase Cloud Messaging (FCM) when our application is in the foreground. When a new message is received, this checks if the message contains a notification payload. If it does, it creates a SnackBar to be displayed on screen.
The content of the SnackBar is shown a Listile and is created with the notification’s title and body as the content.
Testing
Goto Notification composer on firebase console and create a new campaign, and type the notification title and notification text.
Press ‘send test message’ and enter the token printed in the terminal, and press’ ‘Test’’ button.
Now, the foreground notification will be shown on the screen on application.
Want to learn how to use Firebase Analytics?
Follow this next article where we teach you how to add firebase analytics to this application.
ARTICLE LINK: HERE
Conclusion
Today you learned how easy it is to build your very own Flutter Application to which we can send notifications using the firebase console using a token.
You first started out with a default flutter project, then you learned about initializing the basic firebase project and finally we learned how to show and handle foreground and background notifications on screen.
If this article has helped in any way and you have learned something new today, Kindly consider sharing the article so others can also benefit and learn how to build simple AI applications.
Subscribe to our website for more interesting articles on AI and other interesting fields of IT.
You can find the code for this here:
Repository Link:
- Repo link: github
Faheem Ahmed