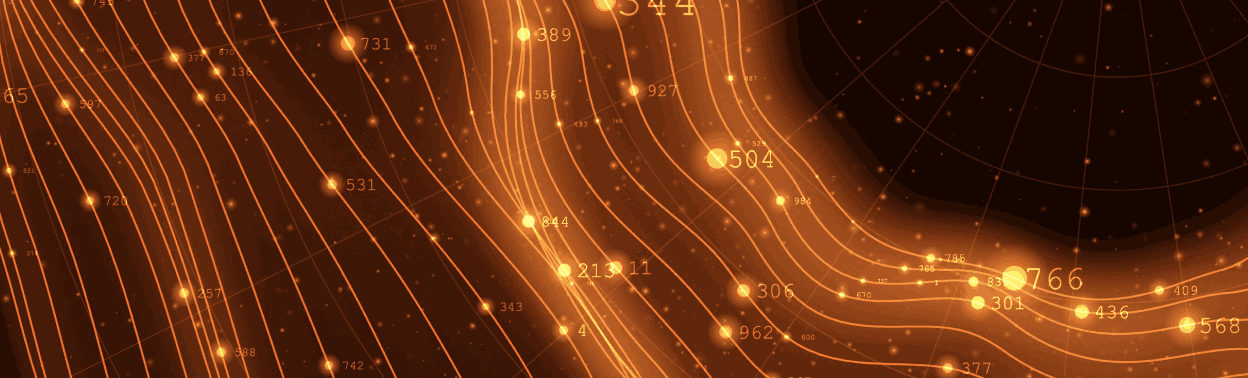
Selenium, the leading and most used open-source framework for testing web and mobile applications, offers various capabilities that cater to various testing needs. However, with its popularity among software engineers, it's crucial to address exceptions that commonly arise while working with implicit wait and explicit wait in selenium.
Therefore, this article focuses on mastering the use of Implicit Wait and Explicit Wait in Selenium, which is essential for effectively handling dynamically loading elements in web applications. By understanding and implementing these commands, QA engineers can not only optimize script execution but also minimize exceptions, ultimately achieving reliable and accurate test results.
Waits in Selenium: Understanding Implicit Wait and Explicit Wait in Selenium
Selenium provides three primary waiting methods for elements: implicit, explicit, and fluent. In this article, we will see the differences between these types of waits, their syntax, and their usage, and understand their advantages and disadvantages while using Selenium webdriver.
Web applications often have elements that load or appear dynamically, requiring test scripts to pause or wait before interacting with them. By understanding implicit wait and explicit wait in selenium and implementing the appropriate Wait commands, engineers can synchronize their test scripts with the application's behavior, leading to more robust and stable automation efforts.
1. Implicit wait
Implicit wait sets a global timeout to be applied to all subsequent web element searches. It instructs the WebDriver to wait a specified time before raising a "NoSuchElementException" if an element cannot be located. The default time is 0.
Once we set the time, the web driver will wait for the element for that time before throwing an exception. The implicit wait is useful when you have a consistent page loading time across your application and want to avoid adding explicit wait statements for every element interaction.
Setting up implicit wait
We can use the implicitly_wait() method to set an implicit wait. The method takes a single parameter: the time in seconds for the WebDriver to wait. Here's an example of setting an implicit wait in a different programming language.
Complete code
Python
# import selenium libraries
from selenium import webdriver
from Selenium.webdriver.common.by import By
from Selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
# Creating a new instance of the Chrome WebDriver using the ChromeDriver manager
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
# Opening the Google homepage
driver.get("https://google.com")
# Maximizing the browser window
driver.maximize_window()
# Setting the implicit wait time to 10 seconds
driver.implicitly_wait(10)
# Finding the element with and inputting data on it
driver.find_element(By.NAME, "q").send_keys("Implicit wait")
Java
import java.util.concurrent.TimeUnit;
import org.openqa. Selenium.WebDriver;
import org.openqa. Selenium.chrome.ChromeDriver;
import org.openqa. Selenium.chrome.ChromeOptions;
import org.openqa. Selenium.By;
import org.openqa. Selenium.WebElement;
import io.github.bonigarcia.wdm.WebDriverManager;
public class ImplicitWaitExample {
public static void main(String[] args) {
// Set up ChromeDriver using WebDriverManager
WebDriverManager.chromedriver().setup();
// Create ChromeOptions object
ChromeOptions options = new ChromeOptions();
// Create a new instance of ChromeDriver
WebDriver driver = new ChromeDriver(options);
// Open the Google homepage
driver.get("https://google.com");
// Maximise the browser window
driver.manage().window().maximize();
// Set the implicit wait time to 10 seconds
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Find the element and input data into it
WebElement element = driver.findElement(By.name("q"));
element.sendKeys("Implicit wait");
// Close the browser
driver.quit(); }}
2. Explicit wait
Explicit wait allows you to wait for a certain condition to occur before proceeding with the execution of your code. It provides more precise control over waiting times and conditions than implicit wait. The explicit wait is more intelligent but can only be applied to specified elements. With explicit wait, you can wait for specific elements to be present, visible, clickable, or have specific attributes.
This is particularly useful when dealing with dynamic web pages or waiting for elements to load before interacting with them. To utilize explicit wait in Selenium, one must have to use ExpectedConditions. The ExpectedConditions class provides a variety of conditions that can be used for explicit waits. Some of the explicit wait functions are as follows:
- presence_of_element_located: Waits for the presence of an element in the DOM.
- visibility_of_element_located: Waits for an element to be visible on the page.
- element_to_be_clickable: Waits for an element to be clickable.
- element_to_be_selected: Waits for an element to be selected.
- text_to_be_present_in_element: Waits for specific text to be present in an element.
- title_contains: Waits for the page title to contain a specific text.
- frame_to_be_available_and_switch_to_it: Waits for a frame & switches the driver’s focus to it.
- alert_is_present: Waits for an alert dialog to be present.
Using Explicit Wait
To use an explicit wait in Selenium, import the WebDriverWait and expected_conditions classes. The WebDriverWait class provides a constructor that takes two parameters: the WebDriver instance and the maximum time to wait. The expected_conditions class provides various predefined conditions to use for your wait. Here's an example of using explicit wait:
Complete code
Python
from selenium import webdriver
from Selenium.webdriver.common.by import By
from Selenium.webdriver.support.ui import WebDriverWait
from Selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Chrome()
driver.get("https://www.google.com")
wait = WebDriverWait(driver, 10) # wait for up to 10 seconds
element = wait.until(EC.presence_of_element_located((By.NAME, “q”)))
Java
import org.openqa. Selenium.By;
import org.openqa. Selenium.WebDriver;
import org.openqa. Selenium.WebElement;
import org.openqa. Selenium.chrome.ChromeDriver;
import org.openqa. Selenium.support.ui.ExpectedConditions;
import org.openqa. Selenium.support.ui.WebDriverWait;
public class SeleniumExample {
public static void main(String[] args) {
// Set up ChromeDriver using WebDriverManager
WebDriverManager.chromedriver().setup();
// Create ChromeOptions object
ChromeOptions options = new ChromeOptions();
// Create a new instance of ChromeDriver
WebDriver driver = new ChromeDriver(options);
// Create a WebDriverWait instance with a timeout of 10 seconds
WebDriverWait wait = new WebDriverWait(driver, 10);
// Open the desired URL
driver.get("https://www.google.com");
// Perform the explicit wait for the presence of the element with name "q"
WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(By.name("q")));
// Perform further actions on the element if needed
// For example, you can send keys to the input field:
element.sendKeys("search query");
// Close the browser and quit the WebDriver instance
driver.quit();
Best Practices for Using Implicit Wait and Explicit Wait in Selenium
To ensure optimized, best test automation performance, and maintainability, follow these best practices when using implicit wait and explicit wait in selenium:
- Avoid using implicit wait whenever possible, as it is inflexible and can lead to longer test execution times.
- Use explicit wait for most cases, providing greater flexibility and support for complex wait conditions.
- Explicit waits provide different functions so they can be used with the specific requirement of the test case.
- Use fluent wait when you need more control over polling intervals or want to ignore specific exceptions.
- Always set a reasonable maximum wait time to prevent tests from waiting indefinitely in case of application errors or unexpected behavior.
- Use descriptive variable names for your wait objects to improve code readability and maintainability.
- Review and update your waiting regularly. Elements and conditions may change. Review and update your waits regularly to ensure they stay effective.
Conclusion
Selecting the right wait type depends on the specific requirements of the test scenario. Understanding implicit and explicit waits in Selenium WebDriver is critical for successful test automation. Understanding their difference is equally vital.
Implicit waits can be useful when elements consistently appear after a certain time. Explicit waits are ideal for waiting for dynamic elements or specific conditions. By following best practices, you may construct more structured, sustainable, and reliable application test scripts.
AIM Digital Technologies Quality Assurance Services
Supercharge your software testing with AIM Digital Technologies! Experience the power of Selenium Waits and automation testing to ensure flawless performance and unparalleled efficiency. Our expert Quality Assurance services harness the full potential of Selenium Waits, allowing you to automate your testing processes and achieve lightning-fast results. Contact us now to embark on a journey of cutting-edge testing solutions! Want to stay updated on the latest advancements in Selenium WebDriver and test automation techniques? Subscribe to our newsletter and receive regular insights, tips, and industry news delivered straight to your inbox. Stay ahead of the curve – subscribe now!
Related Blogs: